How to apply forces
Steps to apply external forces
The steps to apply an external force to a 3D character are summarized below:
- Enable the character's kinetic behavior
- Compute an external force
- Apply the external force
Step 1. Enable Kinetic behavior
Enable the character's kinetic behavior using the method U4DEngine::
//Line 3. Enable Kinetics Behavior myAstronaut->enableKineticsBehavior(); //Line 4. Set mass of entity myAstronaut->initMass(1.0);
Step 2. Compute an External Force
Next, we need to compute the external force and apply it to the model using the method U4DEngine::
void Earth::update(double dt){ //Line 8. Compute a force=m*(vf-vi)/dt //Line 8a. Get the mass of the entity float mass=myAstronaut->getMass(); //Line 8b. set a final velocity vector U4DEngine::U4DVector3n finalVelocity(2.0,0.0,0.0); //Line 8c. compute force U4DEngine::U4DVector3n force=finalVelocity*mass/dt; //Line 9. Apply force to entity myAstronaut->addForce(force); //Line 10. set initial velocity to zero U4DVector3n zero(0.0,0.0,0.0); myAstronaut->setVelocity(zero); }
In line 8a we get the mass of the entity using the method U4DEngine::
In line 9, we apply the force to the 3D entity using the method U4DEngine::
Since we want a constant force, we set the initial velocity to zero as shown in line 10 using the method U4DEngine::
(Optional) Remove Gravity Forces
To prevent entities from falling, the Untold Engine allows you to control the force of gravity through the method U4DEngine::
//Line 5. Set gravity to zero U4DEngine::U4DVector3n zero(0.0,0.0,0.0); myAstronaut->setGravity(zero);
Result
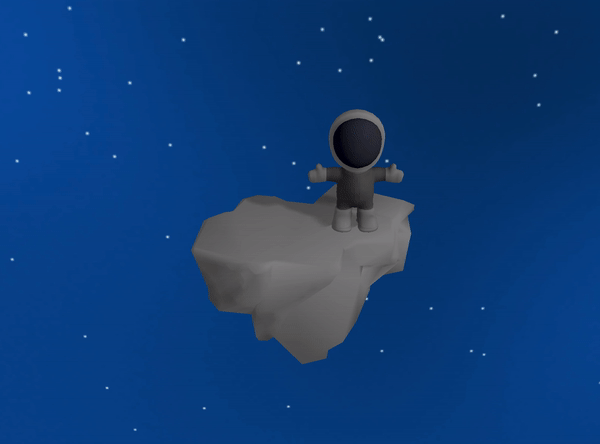
Helper Functions
Here are some code snippets for your reference:
This snippet applies the appropriate force by providing the desired magnitude of the final velocity as an argument.
Note that in this instance, it is required to provide a force direction.
void Astronaut::applyForce(float uFinalVelocity, double dt){ //force=m*(vf-vi)/dt //get the force direction forceDirection.normalize(); //get mass float mass=getMass(); //calculate force U4DEngine::U4DVector3n force=(forceDirection*uFinalVelocity*mass)/dt; //apply force to the character addForce(force); //set inital velocity to zero U4DEngine::U4DVector3n zero(0.0,0.0,0.0); setVelocity(zero); }
Whereas this code snippet applies a force by providing the desired final velocity vector as an argument:
void Astronaut::applyVelocity(U4DEngine::U4DVector3n &uFinalVelocity, double dt){ //force=m*(vf-vi)/dt //get mass float mass=getMass(); //calculate force U4DEngine::U4DVector3n force=(uFinalVelocity*mass)/dt; //apply force to the character addForce(force); //set inital velocity to zero U4DEngine::U4DVector3n zero(0.0,0.0,0.0); setVelocity(zero); }