How to setup camera behavior
The Untold Engine provides several types of camera, such as a First Person Camera, Third Person Camera and a Basic Follow Camera.
Third Person Camera
To implement a Third Person Camera, you need to create Camera of type U4DEngine::
//Instantiate the camera U4DEngine::U4DCamera *camera=U4DEngine::U4DCamera::sharedInstance(); //Line 1. Instantiate the camera interface and the type of camera you desire U4DEngine::U4DCameraInterface *cameraThirdPerson=U4DEngine::U4DCameraThirdPerson::sharedInstance(); //Line 2. Set the parameters for the camera. Such as which model the camera will target, and the offset positions cameraThirdPerson->setParameters(astronaut,0.0,5.0,10.0); //Line 3. set the camera behavior camera->setCameraBehavior(cameraThirdPerson);
The Untold Engine will create a Third Person camera as shown below:
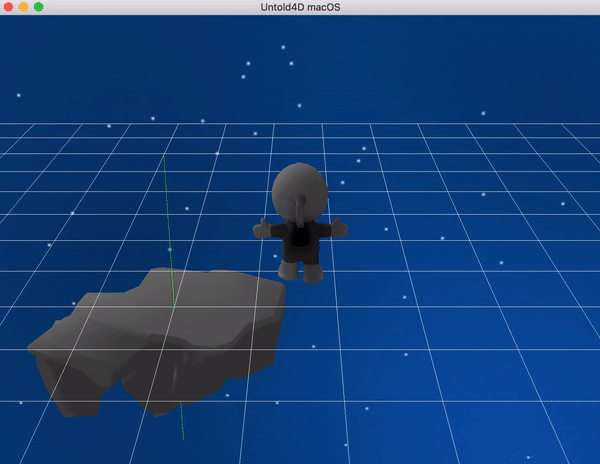
First Person Camera
To implement a First Person Camera, you need to create Camera of type U4DEngine::
Once the Camera Type has been created, you attach the behavior to the Camera object as shown in line 3.
Note that the z-offset position represents the z-axis distance in front of the target 3D model.
//Instantiate the camera U4DEngine::U4DCamera *camera=U4DEngine::U4DCamera::sharedInstance(); //Line 1. Instantiate the camera interface and the type of camera you desire U4DEngine::U4DCameraInterface *cameraFirstPerson=U4DEngine::U4DCameraFirstPerson::sharedInstance(); //Line 2. Set the parameters for the camera. Such as which model the camera will target, and the offset positions cameraFirstPerson->setParameters(astronaut,0.0,0.5,0.5); //Line 3. set the camera behavior camera->setCameraBehavior(cameraFirstPerson);
The Untold Engine will create a First Person camera as shown below:
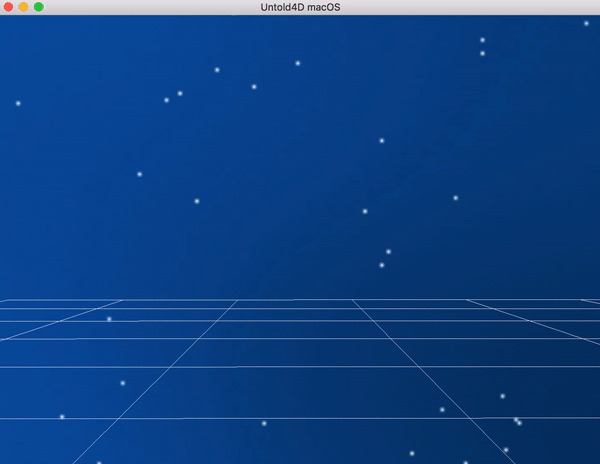
Basic Follow
To implement a Third Person Camera, you need to create Camera of type U4DEngine::
Once the Camera Type has been created, you attach the behavior to the Camera object as shown in line 3.
Note that the Basic Follow Camera does not rotate whenever the target 3D model rotates. It only follows it across the screen.
//Instantiate the camera U4DEngine::U4DCamera *camera=U4DEngine::U4DCamera::sharedInstance(); //Instantiate the camera interface and the type of camera you desire U4DEngine::U4DCameraInterface *cameraBasicFollow=U4DEngine::U4DCameraBasicFollow::sharedInstance(); //Set the parameters for the camera. Such as which model the camera will target, and the offset positions cameraBasicFollow->setParameters(astronaut,0.0,5.0,-10.0); //set the camera behavior camera->setCameraBehavior(cameraBasicFollow);
The Untold Engine will create a Basic Follow camera as shown below:
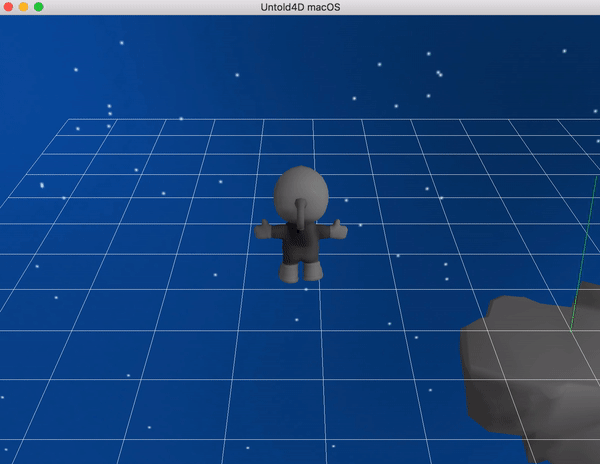