How to enable a game controller
Aside from using the keyboard and mouse, you can also use a game controller. Currently, the game controller supported by the Untold Engine is the Steelseries Nimbus controller.
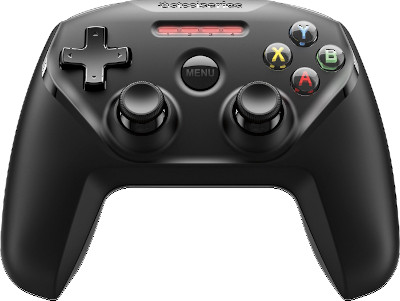
For your convienience, the Untold Engine provides several files that you can use as a reference. Two of these files that you should refer to while enabling the game controller are:
- GameViewController.mm
- GamePadController.mm
To enable the controller, set the following method to true in the viewDidLoad method in the GameViewController file:
//If using the keyboard, then set it to false. If using a controller then set it to true director->setGamePadControllerPresent(true);
The GamePadController class will be enabled once the engine detects the controller. This class will notify the engine whenever you press a button or move the joystick.
The snippet below shows how you can rotate a character by moving the joystick.
//...other actions here // Action detected on mouse case U4DEngine::padRightThumbstick: if(controllerInputMessage.inputElementAction==U4DEngine::padThumbstickMoved){ //Get the joystick position/directions U4DEngine::U4DVector3n joystickDirection(controllerInputMessage.joystickDirection.x,controllerInputMessage.joystickDirection.y,0.0); //The following snippet will determine which way to rotate the model depending on the motion of the thumbstick float joystickMovMagnitude=joystickDirection.magnitude(); joystickDirection.normalize(); U4DEngine::U4DVector3n axis; U4DEngine::U4DVector3n upVector(0.0,1.0,0.0); U4DEngine::U4DVector3n xVector(1.0,0.0,0.0); //get the dot product float upDot, xDot; upDot=joystickDirection.dot(upVector); xDot=joystickDirection.dot(xVector); U4DEngine::U4DVector3n v=pPlayer->getViewInDirection(); v.normalize(); //if direction is closest to upvector if(std::abs(upDot)>=std::abs(xDot)){ //rotate about x axis // if(upDot>0.0){ // // axis=v.cross(upVector); // // }else{ // axis=v.cross(upVector)*-1.0; // // } }else{ //rotate about y axis if(xDot>0.0){ axis=upVector; }else{ axis=upVector*-1.0; } } //Once we know the angle and axis of rotation, we can rotate the model using interpolation as shown below float angle=1.0*joystickMovMagnitude; float biasAngleAccumulator=0.90; angleAccumulator=angleAccumulator*biasAngleAccumulator+angle*(1.0-biasAngleAccumulator); U4DEngine::U4DQuaternion newOrientation(angleAccumulator,axis); U4DEngine::U4DQuaternion modelOrientation=pPlayer->getAbsoluteSpaceOrientation(); U4DEngine::U4DQuaternion p=modelOrientation.slerp(newOrientation,1.0); pPlayer->rotateBy(p); }else if (controllerInputMessage.inputElementAction==U4DEngine::padThumbstickReleased){ } break;