How to enable the Follow-Path Steering Behavior
The Follow Path Steering Behavior returns the final velocity that directs the agent to follow a defined path.
The image below shows the process to compute the Target Position and consequently compute the final velocity required to direct the agent along the path.
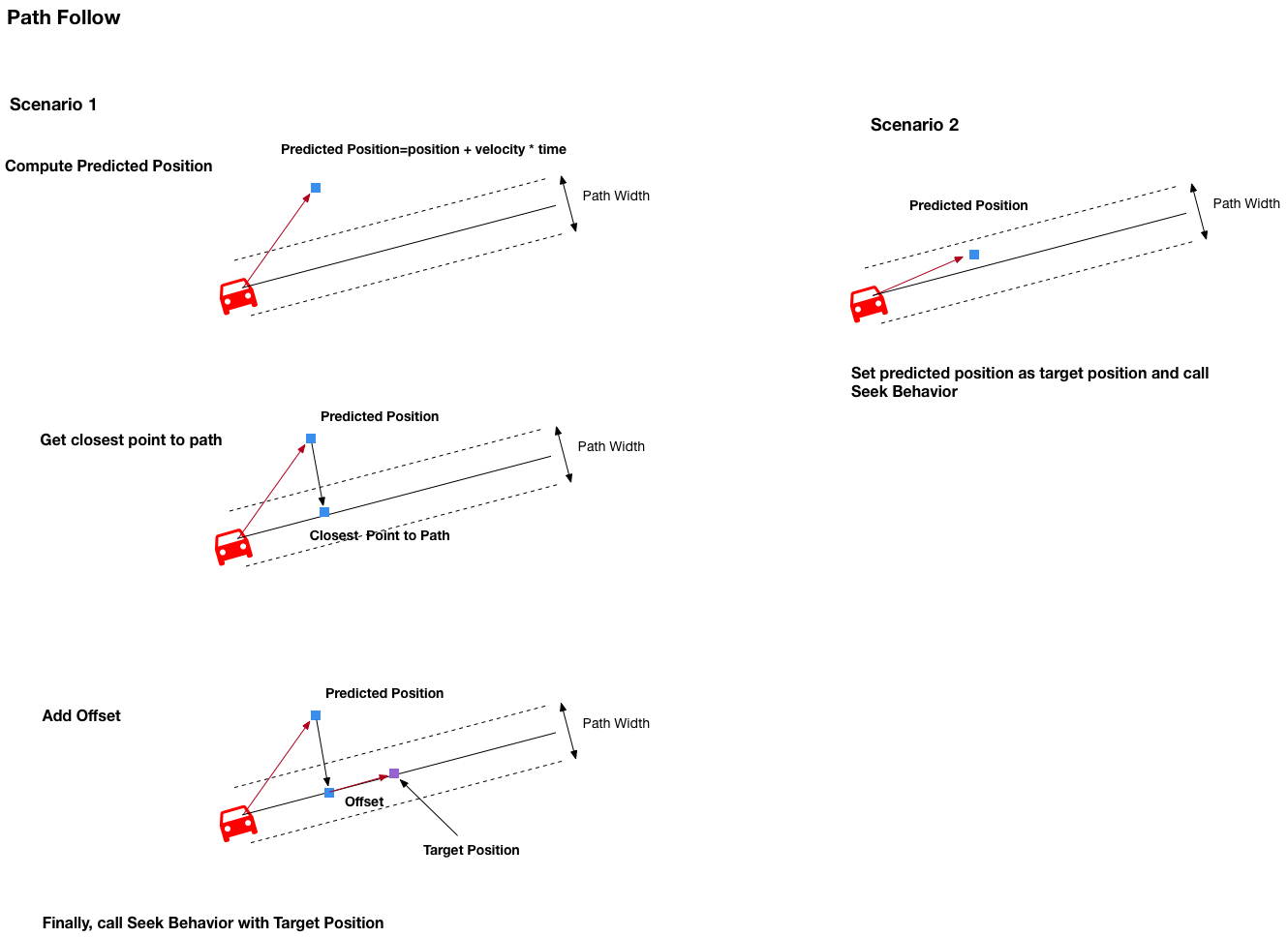
You can set the time used to predict the agent's position by using:
void setPredictTime(float uPredictTime)
You can also set the path Offset. This is the offset distance along the path to generate the target position by using: void setPathOffset(float uPathOffset)
The path radius/width can be defined by using:
void setPathRadius(float uPathRadius)
The code snippet below shows how to compute Follow Path final velocity.
//1. Create an instance of the Follow Path object U4DEngine::U4DFollowPath *followPathBehavior=new U4DEngine::U4DFollowPath(); //2. Set the time in the future to predict the position followPathBehavior->setPredictTime(0.5); //3. Set the distance along the path to generate the target position followPathBehavior->setPathOffset(0.3); //4. Set the path radius followPathBehavior->setPathRadius(0.5); //5. Generate the segment data. To compute the the final velocity, the U4DFollowPath object requires the segment data. U4DEngine::U4DPoint3n a(0.09,0.51,-0.38); U4DEngine::U4DPoint3n b(1.47,0.51,-1.18); U4DEngine::U4DPoint3n c(2.77,0.51,0.176); U4DEngine::U4DPoint3n d(4.66,0.51,-0.263); U4DEngine::U4DPoint3n e(7.30,0.51,-2.80); U4DEngine::U4DSegment segmentA(a,b); U4DEngine::U4DSegment segmentB(b,c); U4DEngine::U4DSegment segmentC(c,d); U4DEngine::U4DSegment segmentD(d,e); //the pathContainer is a vector container std::vector<U4DEngine::U4DSegment> pathContainer; //Push the segment data into the container. pathContainer.push_back(segmentA); pathContainer.push_back(segmentB); pathContainer.push_back(segmentC); pathContainer.push_back(segmentD); //6. Compute the final velocity to steer the agent along the path. The getSteering method requires a pointer to the agent and a vector containing the segmetn data (pathContainer). uSoldier is an instance of your game character. U4DEngine::U4DVector3n finalVelocity=followPathBehavior->getSteering(uSoldier, pathContainer); finalVelocity.y=0.0; //Check if the resulting velocity is set to zero if(!(finalVelocity==U4DEngine::U4DVector3n(0.0,0.0,0.0))){ uSoldier->applyVelocity(finalVelocity, dt); uSoldier->setViewDirection(finalVelocity); }