How to create a camera object
The Untold Engine represents a camera as a U4DEngine::
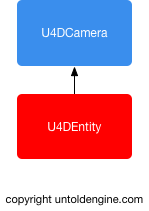
Unlike other entities, a camera is a singleton object. That is, the engine prevents more than one instance of a camera to be created throughout the lifetime of a game.
A camera is created through its singleton method as shown below:
U4DEngine::U4DCamera *camera=U4DEngine::U4DCamera::sharedInstance();
Camera Transformation
A camera inherits its transformation properties from an U4DEntity object. Therefore, it can be translated and rotated.
Translating the camera
A camera can be translated to a particular location by using the translateTo() as shown below:
//Get an instance of the camera U4DEngine::U4DCamera *camera=U4DEngine::U4DCamera::sharedInstance(); //Translate the camera 5.0 units along the y-axis (up) and -10.0 units along the z-axis camera->translateTo(0.0,5.0,-10.0);
The illustrations below show the position of the camera after the translation:
Top View
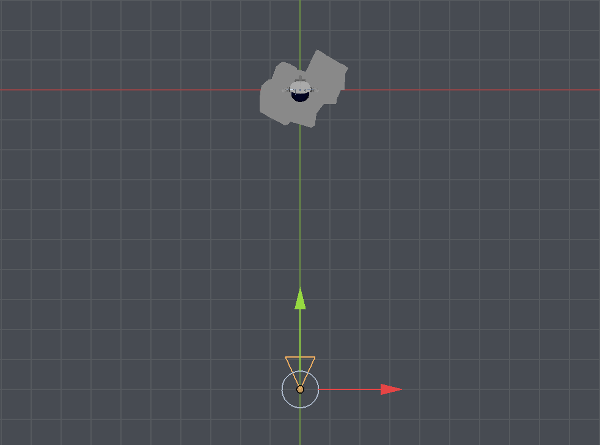
Side View
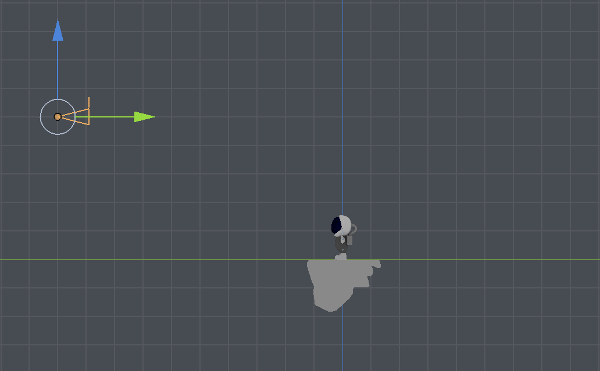
A camera can also be translated by a certain amount using the translateBy() method.
Rotating the camera
A camera can be rotated to a particular angle by using the rotateTo() method, as shown below:
//Get an instance of the camera U4DEngine::U4DCamera *camera=U4DEngine::U4DCamera::sharedInstance(); //Rotate the camera 5.0 degrees about the y-axis camera->rotateTo(0.0,5.0,0.0);
The following illustration shows a top view of the camera after a rotation:
Top View
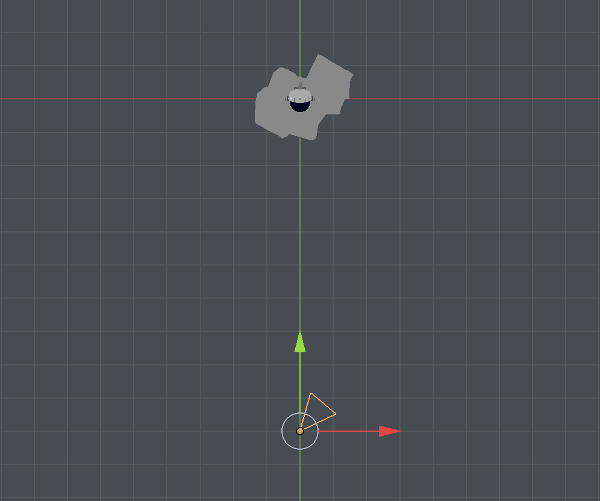
View-in-Direction
There are instances when is necessary to set the camera's view-direction in a particular direction.
The Untold Engine provides the viewInDirection() method, which rotates the camera in a particular direction.
For example, the following snippet shows how to direct the camera's view-direction towards the origin vector.
//set origin point U4DEngine::U4DVector3n origin(0,0,0); //Get an instance of the camera U4DEngine::U4DCamera *camera=U4DEngine::U4DCamera::sharedInstance(); //Translate the camera 5.0 units along the y-axis (up) and -10.0 units along the z-axis camera->translateTo(0.0,5.0,-10.0); //Set the view direction towards the origin vector camera->viewInDirection(origin);
The illustration below shows the camera's view-direction pointing towards the origin vector.
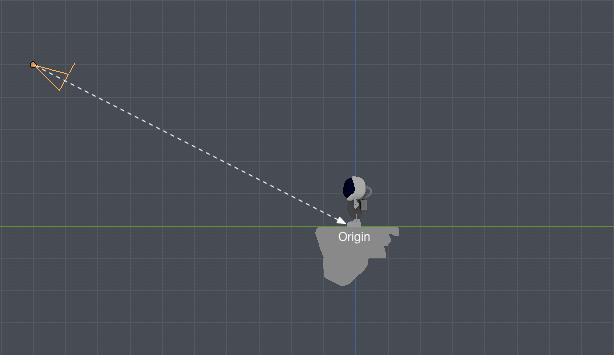