How to animate sprites
The Untold Engine supports sprites animation. The engine represents a sprite animation as a U4DEngine::
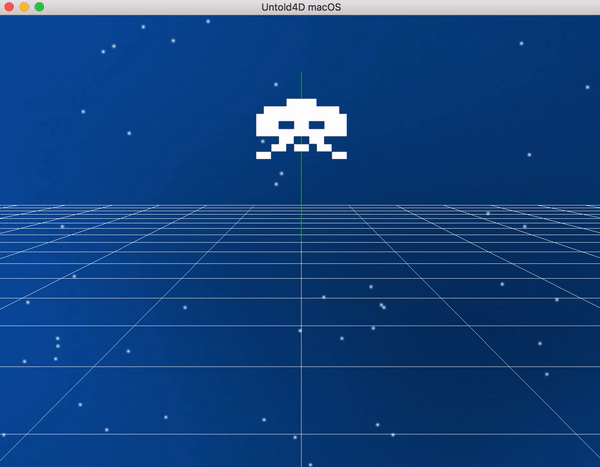
Pre-requisite
A U4DSpriteAnimation object requires that you have successfully created a U4DEngine::
//Line 1. create a a sprite loader object U4DEngine::U4DSpriteLoader *spriteLoader=new U4DEngine::U4DSpriteLoader(); //Line 2. load the sprite information into the loader spriteLoader->loadSpritesAssetFile("spaceinvaderspritesheet.xml", "spaceinvaderspritesheet.png"); //Line 3. Create a sprite object U4DEngine::U4DSprite *mySprite=new U4DEngine::U4DSprite(spriteLoader); //Line 4. Set the sprite to render mySprite->setSprite("spaceinvaderoctopus1.png"); //Line 5.Add it to the world addChild(mySprite,-2); //Line 6. translate the sprite mySprite->translateTo(0.0,0.5,0.0);
Step 1. Load animation sprites
Once a spritesheet has been loaded into a U4DEngine::
//Line 7. Load all the sprite animation data into the structure U4DEngine::SPRITEANIMATIONDATA spriteAnimationData; spriteAnimationData.animationSprites.push_back("spaceinvaderoctopus1.png"); spriteAnimationData.animationSprites.push_back("spaceinvaderoctopus2.png"); //Line 8. set a delay for the animation spriteAnimationData.delay=0.2;
In this example, the sprites that will be part of the animation are:
- spaceinvaderoctopus1.png
- spaceinvaderoctopus2.png
These sprites are highlighted in the spritesheet below:
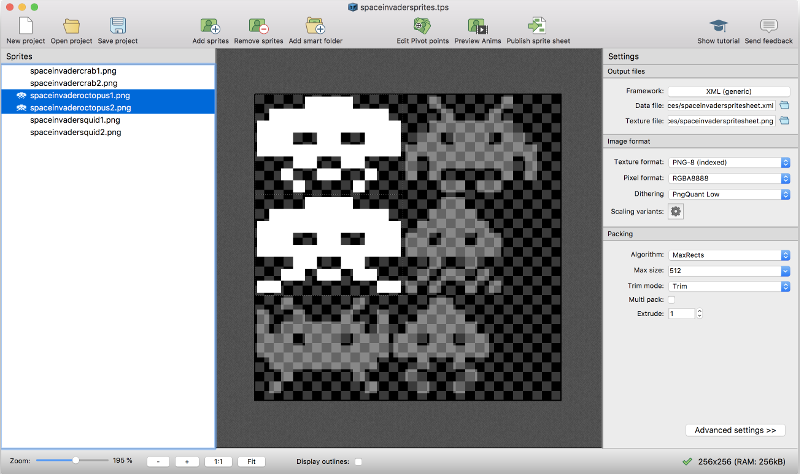
Line 8 provides a delay interval for the animation. Basically, it determines how fast to play the animation.
Create Sprite Animation Object
Once the U4DEngine::
Finally, the sprite animation object is started by calling its play() method, as shown in line 10.
//Line 9. create a sprite animation object U4DEngine::U4DSpriteAnimation *spriteAnim=new U4DEngine::U4DSpriteAnimation(mySprite,spriteAnimationData); //Line 10. Play the sprite animation spriteAnim->play();
Here is the result:
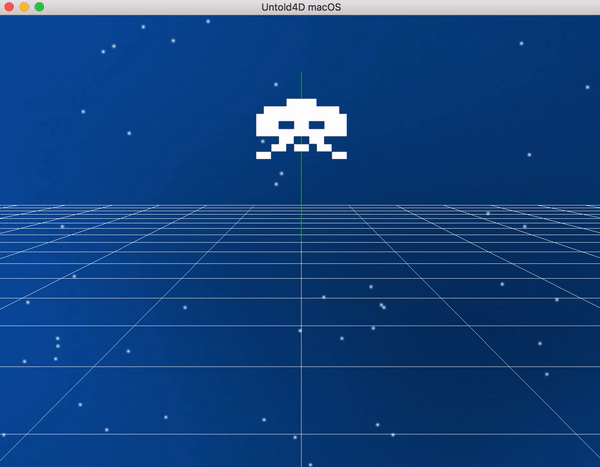