How to translate/rotate a 3D object
Translating a 3D Object
The Untold Engine provides two ways to translate a game object. The engine can translate an object to an absolute position. Or it can translate the object by a specified amount.
For example, imagine the original position of the object is (3,0,0). If you want to move the object to location (5,0,0), you inform the engine to move the entity To position (5,0,0).
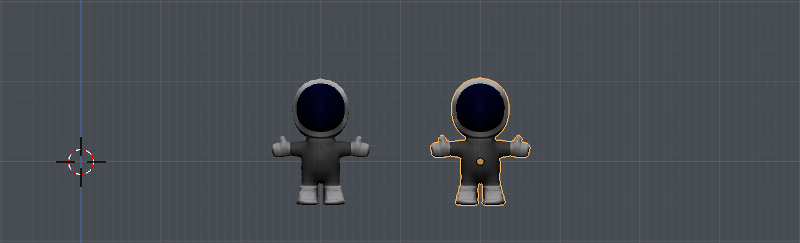
However, if you move the entity By an amount of (5,0,0), the engine will move the object to position (8,0,0).
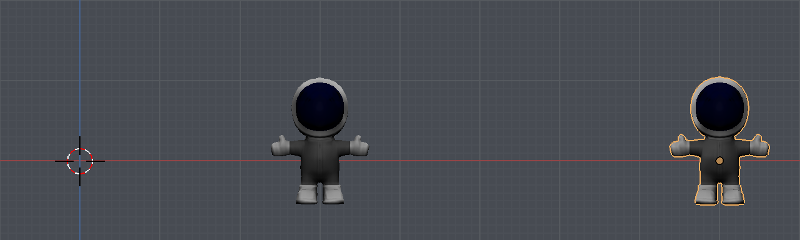
1. Translate To Location
To translate an entity to a location, you use the method U4DEngine::
For example, the snippet below shows how to translate the character to location (-2.0,1.0,2.0).
//Line 5. Translate the game object to location (-2.0, 1.0, 2.0) myAstronaut->translateTo(-2.0, 1.0, 2.0);
2. Translate By an amount
To translate an entity by a particular amount, you use the method U4DEngine::
For example, the snippet below translates the character by 5.0 units along the +x-axis;
//Line 6. Translate the game object BY an amount of 5.0 units along the x-axis myAstronaut->translateBy(5.0, 0.0, 0.0);
Rotating a 3D Object
<iframe width="560" height="315" src="https://www.youtube.com/embed/gLH3dBByTTU" frameborder="0" allow="accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture" allowfullscreen>=""></iframe>
A game character can be rotated To a fixed orientation, or it can be rotated By a certain angle amount.
For example, imagine an entity's initial orientation is 25 degrees about the y-axis.
The entity can rotate To an orientation of 60 degrees about the y-axis, as shown below:
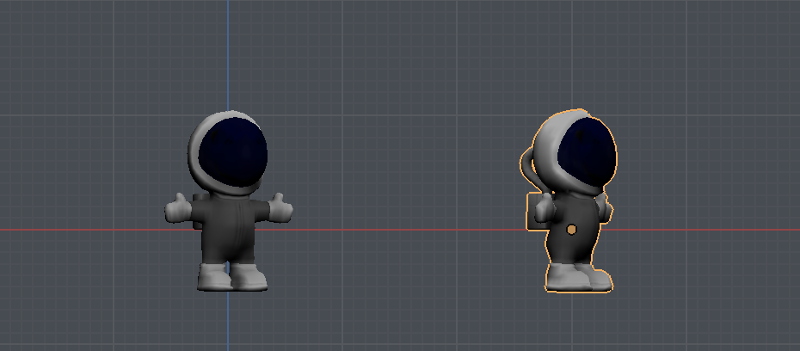
Or it can change its orientation By 60 degree about the y-axis. Doing so will rotate the entity 85 degrees about the y-axis, as shown below:
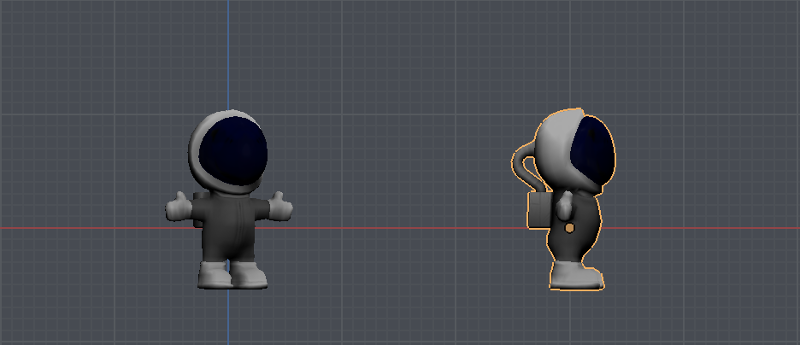
Rotate To an Orientation
To rotate an entity to an orientation of 45 degrees about the y-axis, you use the method U4DEngine::
//Line 5. Rotate the character 45 degress about the y-axis myAstronaut->rotateTo(0.0, 45.0, 0.0);
Rotate By an Amount
To rotate an entiy by an amount of 1.0 degrees about the y-axis, you use the method U4DEngine::
//Line 6. Rotate the character by 1.0 degree about the y-axis myAstronaut->rotateBy(0.0, 1.0, 0.0);
Setting View Direction
A 3D artist is free to model a character as he/she wishes.
For example, The artist can model a character with its default view-direction looking out the screen, as shown below:
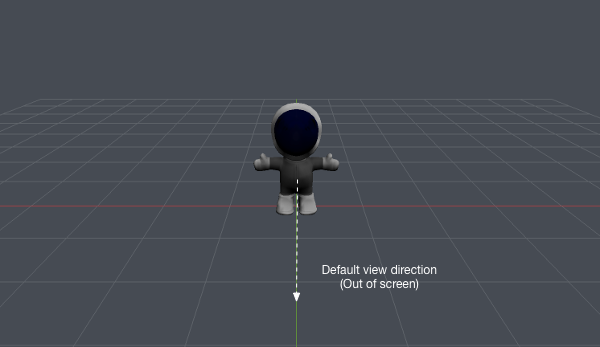
Or can model the character with its default view-direction looking into the screen, as shown below:
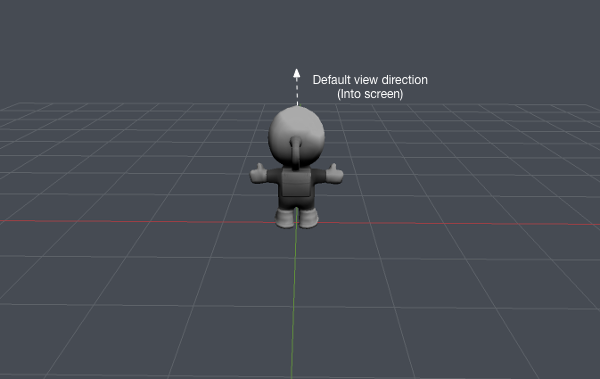
The Untold Engine requires the character's default view direction to properly orient the entity.
Set the Default View Direction
To set the character's default view-direction, the Untold Engine provides the U4DEngine::
For example, if a 3D model was modeled with its default view-direction looking out the screen. Then, per the Untold Engine's coordinate system, the default view-direction corresponds to the vector (0.0,0.0,-1.0).
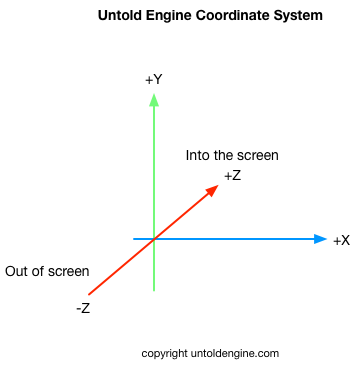
The character's default view-direction would be set as follows:
U4DEngine::U4DVector3n viewDirectionVector(0,0,-1); myAstronaut->setEntityForwardVector(viewDirectionVector);
View-in-Direction
The U4DEngine::
Note: You must specify the default view-direction to properly use this method.
For example, the code below sets the view direction of the astronaut to vector location (-10.0,0.79,10.0):
U4DVector3n viewVector(-10.0,0.79,10.0); myAstronaut->viewInDirection(viewVector);
The engine will orient the astronaut as follows:
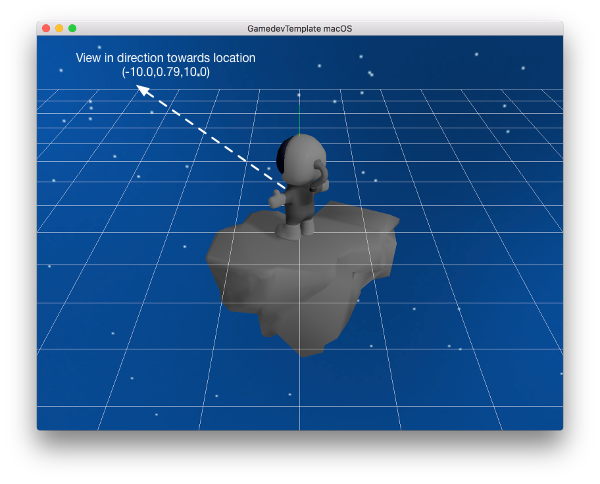
As another example, the code below sets the view direction of the character to (-10.0,0.79,-10.0)
U4DVector3n viewVector(-10.0,0.79,-10.0); myAstronaut->viewInDirection(viewVector);
The engine will orient the astronaut as shown below:
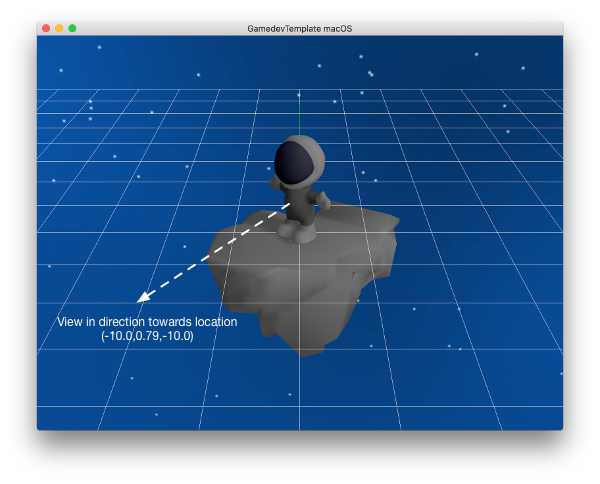